Efficient Document Testing with CenterTest
Documents are your formal communication with your customers, from general information to legal documents. If they are incorrect or not compliant with regulations, you can be at risk financially. If they are unprofessional or incomplete, you can risk your reputation. In any case, documents must be tested. Let’s delve deeper into why automated document testing is hard, and how CenterTest resolves these challenges.
Why Is Document Testing Hard?
There are a few reasons document testing is often not automated, including:
- document context,
- document structure, and
- slow document creation.
Document Context
Unlike code that is logically structured and operates in a predefined manner, human language is intricate and nuanced. Context plays a huge role in understanding and interpreting the content of a document and automated systems often struggle with nuance, tone, and cultural implications.
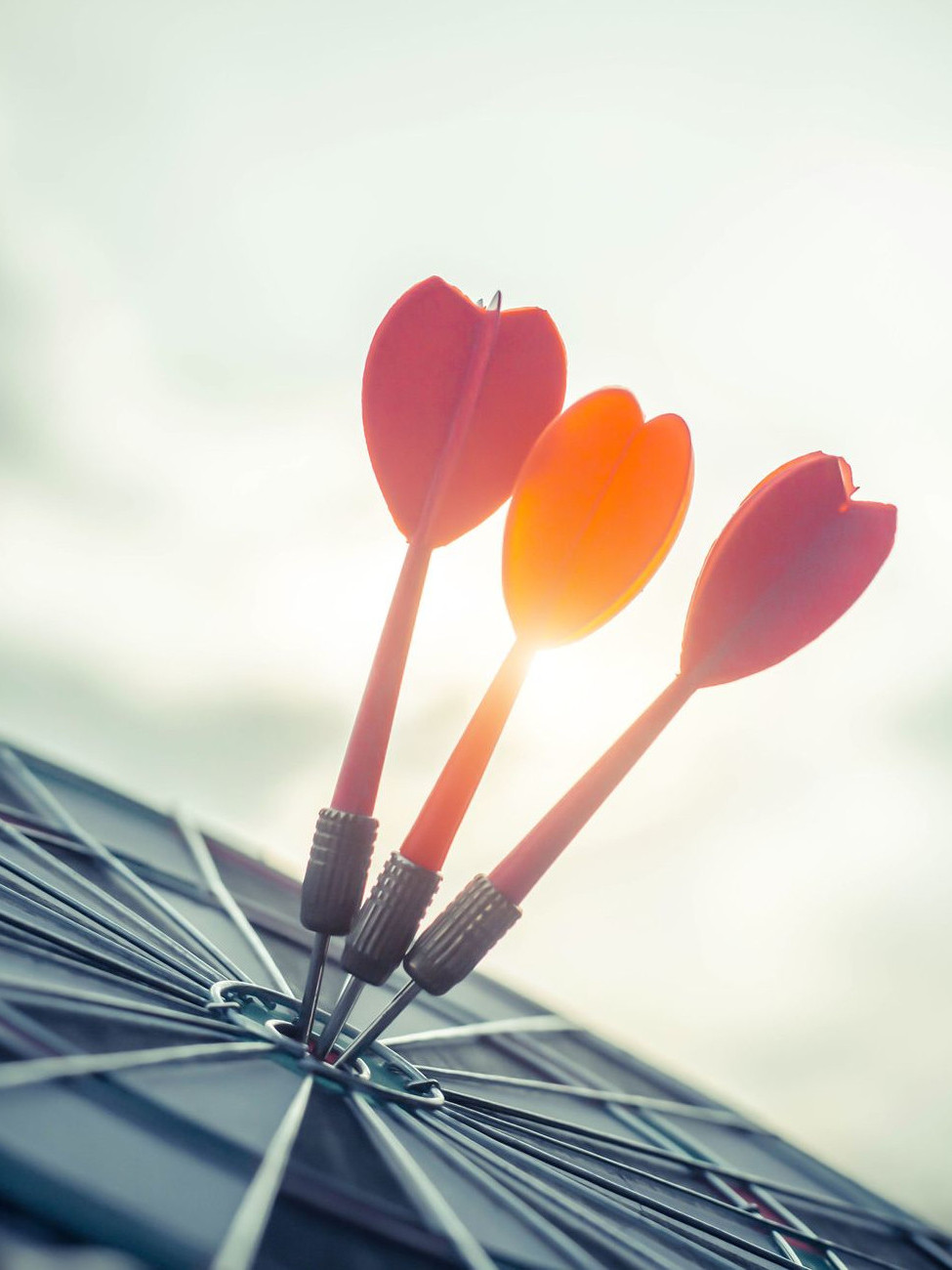
CenterTest addresses this by not pretending to understand the document, but instead verifies the text you tell it to verify. This can account for 95% or more of your document testing needs, making it an easy win. The remaining 5% can still be manually tested, or may not even be part of document testing if they’re verified before being incorporated into the document. For example, legal paragraphs are likely verified before being provided to the document team. In these cases, testing just needs to ensure the paragraph is included versus the content of the paragraph.
Document Structure
As with test automation in general, changes to the structure often break your tests. CenterTest addresses this in two ways: object-oriented principles letting you make changes to a reusable component to correct all tests referring to it, and section validation which lets you find sections even if they move to different locations within the document.
Slow Document Creation
Another common issue with document testing is delays in creating the documents. Test environments often create documents slower than production environments, and create significantly more documents due to testing. These issues combined can result in documents taking minutes to hours longer to generate than production systems.
When manually testing, this isn’t a problem. You just wait an hour, or a day, before validating your document. Effectively, you wait, you open, you validate. CenterTest does the same thing: it waits, then opens, then validates.
How? CenterTest uses self-managed restart technology to wait. When your test generates the document, you simply tell it to open the document using CenterTest utilities.
var doc = new OotbDocumentUtilities(getContext(), "Policy.pdf");
var lines = doc.getLines();
The above navigates to the appropriate page to confirm the document is available. If it isn’t, it throws a DocumentNotAvailable exception and fails the test with a restartable error. CenterTest restarts the test automatically after a pre-determined process (the self-managed piece), skips all completed steps until it checks the document again, and repeats this until the document is available. Once available, it is downloaded and opened, and getLines() reads it.
Now that wait and open are addressed, we validate.
Validate the document
Once opened, it’s time to start validating the document. CenterTest does this the same way a person does it, by looking for specific information in specific places in the document.
What to look for is done by simply asserting the text you’re looking for. For example, the following code looks for the string anywhere within the document, or you can find a label and verify the text related to that label. If found, the assert passes. If not, the assert fails with a “soft assertion”, which we’ll talk about a little later.
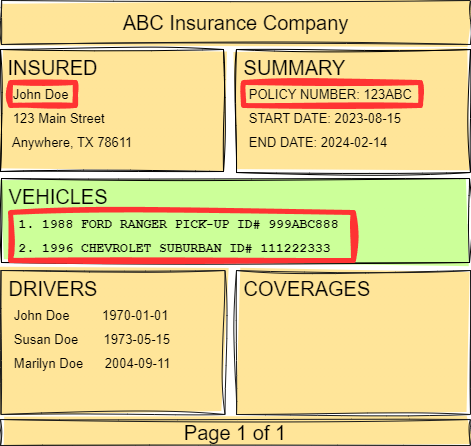
doc.assertNow(assertions, lines, "John Doe");
doc.assertNow(assertions, lines, "Policy Number:", "123ABC");
Where to look is within the entire document by default. You can also identify a specific section within a document to validate. This is done by identifying a portion of the document between a starting text and an ending text. For example, the VEHICLES section in green above.
var vehicles = getSection("VEHICLES", "DRIVERS")
This looks for “VEHICLES”and marks the starting point of a section after the text. It then looks for “DRIVERS” to identify the end of the section. Once identified, you can search for text within a section, similar to doing simple searches. Let’s see how:
doc.assertNow(assertions, vehicles, "1988 FORD RANGER PICK-UP ID# 999ABC888");
doc.assertNow(assertions, vehicles, "1996 CHEVROLET SUBURBAN ID# 111222333");
In a simple search, the document is searched (“lines”). In a section search, a “vehicles” section is identified and that is searched. As simple as that!
What are Soft Assertions?
Asserts generally stop testing when any assert fails. This is normally good, but since documents don’t change during testing, we want to be efficient and verify the whole document at once. How do we do this? With soft assertions.
Soft assertions are a special JUnit class that collects assert results until you assertAll() to complete the soft assertions.
var assertions = new SoftAssertions();
...
assertions.assertAll();
Once assertAll() is invoked, if any assert has failed, your test fails and returns all the failure messages together at the same time. If there are no failures, your test continues.
What doesn’t CenterTest do?
CenterTest supports all the textual testing you want to do, probably 95% or more of your testing needs. However, there are a couple of things CenterTest doesn’t do: validate images and check grammar.
Putting it all together
Let’s put it all together into a simple test to summarize document testing:
var doc = new OotbDocumentUtilities(getContext(), "Policy.pdf");
var lines = doc.getLines();
var assertions = new SoftAssertions();
doc.assertNow(assertions, lines, "POLICY NUMBER: 123ABC123ABC");
doc.assertNow(assertions, lines, "START DATE", "2023-08-15");
doc.assertNow(assertions, lines, "END DATE", "2024-02-14");
var insured = getSection("INSURED", "SUMMARY");
doc.assertNow(assertions, insured, "John Doe");
doc.assertNow(assertions, insured, "123 Main Street");
doc.assertNow(assertions, insured, "Anywhere, TX 78611");
var vehicles = getSection("VEHICLES", "DRIVERS")
doc.assertNow(assertions, declarations, "1988 FORD RANGER PICK-UP ID#123ABC456");
doc.assertNow(assertions, declarations, "1996 CHEVROLET SUBURBAN ID#456DEF789");
assertions.assertAll();
This test does all three necessary steps for automated document validation: wait, open and validate.
- Self-managed restarts ensure your test waits for the document
- DocumentUtilities then downloads and opens the document
- Soft asserts are used to validate the document all at once
CenterTest makes it easy to incorporate document testing into your CI/CD process. Start document testing today using CenterTest, and eliminate all the unnecessary time spent manually testing documents!
Kim Filiatrault
Founder and President
Watch or read our other posts at Kimputing Blogs. You’ll find everything from Automated testing to CenterTest, Guidewire knowledge to general interest. We’re trying to help share our knowledge from decades of experience.